Debugging and Implementing the Undo Feature
This is the continuing story of using OpenAI’s ChatGPT to build a turn-based strategy game. I started with the idea of building something like a game called Anityoy, which I enjoy. The process is evolving and gets off to a rocky start in Part 1. The object is to get ChatGPT to write all the code, which is a debugging process unique to AI-assisted game development, code optimization, and working with OpenAI ChatGPT.
In this session, I wanted to complete the tasks I started in Part 2, namely get the functions into classes and have working code.
In Part 2 Chat Version 4 hit a usage limitation and I took the option to move to version 3.5 in the same session. This did not go as well as I hoped. For this session I began an entirely New Chat in version 4 with the following prompt:
You are helping me create a turn-based strategy game. You have given me some code. It has errors. I will supply the code and you will help me fix it. There are two code files.
I included the code for the two files in the prompt.
This was the right choice. Chat found the issues with the mousePressed() function and provided a new, complete function. A fix to the endTurn(), isValidMove() and one more update to the mousePressed() functions got the code working again without error. I have gotten to a point where I am not asking to fix errors but add or improve functionality. This feels like progress.
One important feature is to have an Undo method. This was working in Part 1 and broke after the changes in Part 2. Like all changes, iterating with Chat is not ask once and gain perfection. It is ask once, get something working, and break two other things, then ask again and again. Here is an example of one exchange, and with the apologies version 4 provides.
This is partially working. After a player uses the Undo method, a new move cannot be made. When a move is undone, the Unit should be able to move again.
I apologize for the confusion. I see the issue now - the hasMoved property of the unit is not being reset when the move is undone. Let's update the undo() function to reset the hasMoved property of the unit when a move is undone.
function undo() {
if (moveHistory.length > 0) {
lastAction = moveHistory.pop(); // Get the last move from moveHistory
if (lastAction.type === "move") {
let { fromX, fromY, toX, toY, fromCell, toCell } = lastAction;
// Undo the move
boardInstance.grid[fromY][fromX] = Object.assign(new Tile(), fromCell);
boardInstance.grid[toY][toX] = Object.assign(new Tile(), toCell);
// Reassign the unit object to the corresponding tile
if (fromCell.unit) {
fromCell.unit.tile = boardInstance.grid[fromY][fromX];
fromCell.unit.hasMoved = false; // Reset the hasMoved property
}
if (toCell.unit) {
toCell.unit.tile = boardInstance.grid[toY][toX];
}
selectedUnit = null;
redraw();
}
}
}
Now, when a move is undone, the hasMoved property of the unit will be reset, allowing the unit to move again.
The Undo button is working as expected after this. However, a previously fixed issue has reemerged. Tiles a player’s unit moves to should remained ‘owned’ by the player. And so, another round of exchanges begines to resolve the problem. What is interesting is I have not seen any coding errors since the bugs created in Part 2 were addressed.
The fixed and un-fixed cycle is frustrating. I decided I need to make a more direct suggestion to move undo function into the Board class, which puts it on par with the moveUnit() function. Chat provided a list of several changes, noting lines to add and code to insert, but not providing the entire contents of a class or function. This is where errors manifested in Part 1, because it was not always clear where code changes should be made.
This change caused the code to not function as expected – again. I found it helps to give Chat the body of an entire class to have it sort out the issue. I provided the Board class and was told there are several issues to fix. After applying this round of suggestions, I get an actual execution error. The Board.draw() method is calling functions that don’t exist. Rather than give the error message to Chat, I provide the following very descriptive message:
the Board.draw() now throws an error when a player Undo's a move.
I apologize for the oversight. The error occurs because we are attempting to redraw the entire grid after undoing a move. Instead, we should only redraw the specific tiles affected by the undo action. Update the undoMove() function inside the Board class to only redraw the fromCell and toCell after undoing the move
Chat provides yet another updated function definition, and the debugging process continues. I have done enough for the day and will take this on again later. I have some observations.
- I get better results if I can provide the code for ChatGPT 4 to review along with what I am seeing happen.
- Starting a new Chat session is helpful, especially if the session has gotten very lengthy.
- When I started a new session, providing all the relevent code, a description of what the purpose is, and describing the problem helps Chat get to a solution quickly.
- I do not have to write any code (but it helps if you understand code to some extent).
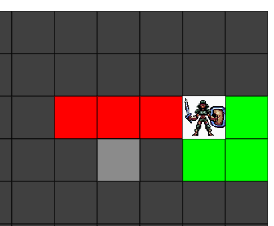
Enough for now. I will get back to this in a day or two.